Introduction of Stripe
If you want to start your online business to sell some products and services. It Quite easy and straightforward to start. There are too many available in the market that can ease your work. The biggest problem for most startups is how to accept the payment for the end-user who is purchasing the product. We have lots of already built-in software in the market that can do this work for you with a secure socket.
Installation
First, install the stripe library in your project using composer. If you have not installed the composer in your system then click here to learn how to install the composer in your system.
composer require stripe/stripe-php
OR
Add the below line in composer.json
"stripe/stripe-php": "^7.60",
Now run the below command
composer install
Account setup on stripe
Create an account on stripe.com using the email id. There you will see two different environment modes first for the test env and second for the live environment. copy your private key from the test env and put it safely.
Note: Change this key from the live when you will go to the production
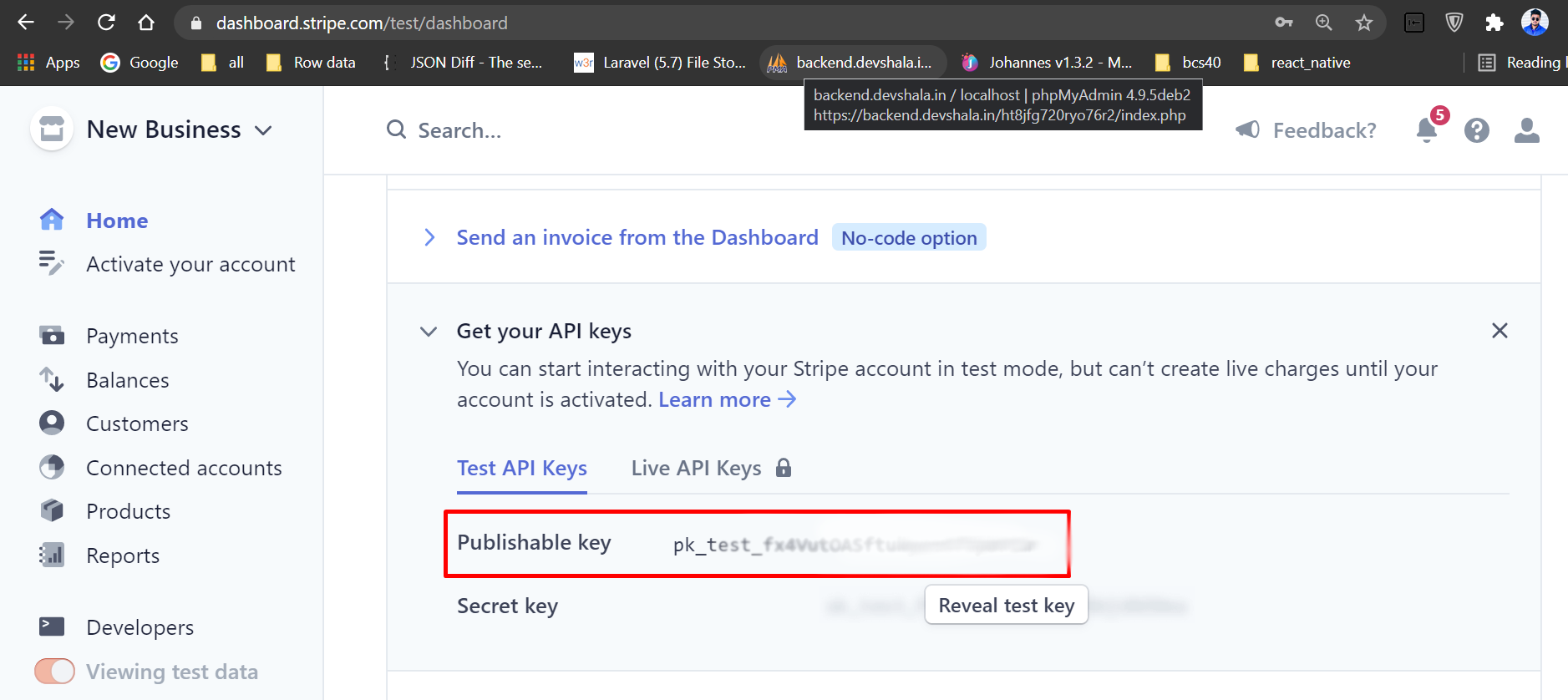
Code Integration
Paste your API key in the below code to connect stripe with your account.
$STRIPE_API_KEY = “replace this text with your private key”; \Stripe\Stripe::setApiKey($STRIPE_API_KEY);
Creating tokens for payment using stripe Token API.
$tokenData = \Stripe\Token::create([ 'card' => [ 'number' => $cardNo, //4242424242424242 'exp_month' => $cardExpMonth, //12 'exp_year' => $cardExpYear, //2021 'cvc' => $cardCvc, //123 ], ]);
Creating the payment using the token which we created above.
$customer = \Stripe\Customer::create(array( 'email' => $email, //demo@gmail.com 'source' => $tokenData, 'name' => $firstName, //Deepak 'address' => [ 'line1' => '510 Townsend St', 'postal_code' => '98140', 'city' => 'San Francisco', 'state' => 'CA', 'country' => 'US', ], ));
Make payment using stripe payment API
$charge = \Stripe\Charge::create(array( 'amount' => $orderTotal, //total amount that will be paid by the user. 'currency' => 'inr', //currency in which he is paying 'customer' => $customer->id, 'description' => 'Test Purchase', //Payment description ));
$charge will return true if the payment has done successfully and false for payment not done successfully.
if($charge){ return “success”; }else{ return “Failed”; }
Dummy card details for the stripe payment gateway
CARD NUMBER | CARD BRAND | CARD CVC | CARD DATE |
4242424242424242 | Visa | Any 3 digits | Any future date |
4000056655665556 | Visa (debit) | Any 3 digits | Any future date |
5555555555554444 | Mastercard | Any 3 digits | Any future date |
2223003122003222 | Mastercard (2-series) | Any 3 digits | Any future date |
Download the complete code file from from here
I Hope the is helpful for you pls share your feedback. That will motivate us more than anything else
Thank you
Dear TechTechInfo, dear David, unfortunately I can’t download the file: http://techtechinfo.com/files/stripePayment.php it shows an 403 error.
Can you please solve that issue for me?
Thank you so far, for this short and clear description.